Hello! In the last lecture, we got acquainted with such an aspect of the Java language as exceptions and saw examples of working with them. Today we will take a deeper look at their structure, and also learn how to write our own exceptions :)
All exceptions have a common ancestor class
All checked exceptions come from the
Types of exceptions
As we said, there are a lot of exception classes in Java, almost 400! But they are all divided into groups, so it’s quite easy to remember them. Here's what it looks like:Throwable
. Two large groups come from it - exceptions (Exception) and errors (Error). Error is a critical error during program execution associated with the operation of the Java virtual machine. In most cases, Error does not need to be handled, since it indicates some serious flaws in the code. The most famous errors: StackOverflowError
- occurs, for example, when a method endlessly calls itself, and OutOfMemoryError
- occurs when there is not enough memory to create new objects. As you can see, in these situations, most often there is nothing special to process - the code is simply written incorrectly and needs to be redone. Exceptions are, in fact, exceptions: an exceptional, unplanned situation that occurred while the program was running. These are not errors as serious as Error, but they require our attention. All exceptions are divided into 2 types - checked ( checked ) and unchecked ( unchecked ). 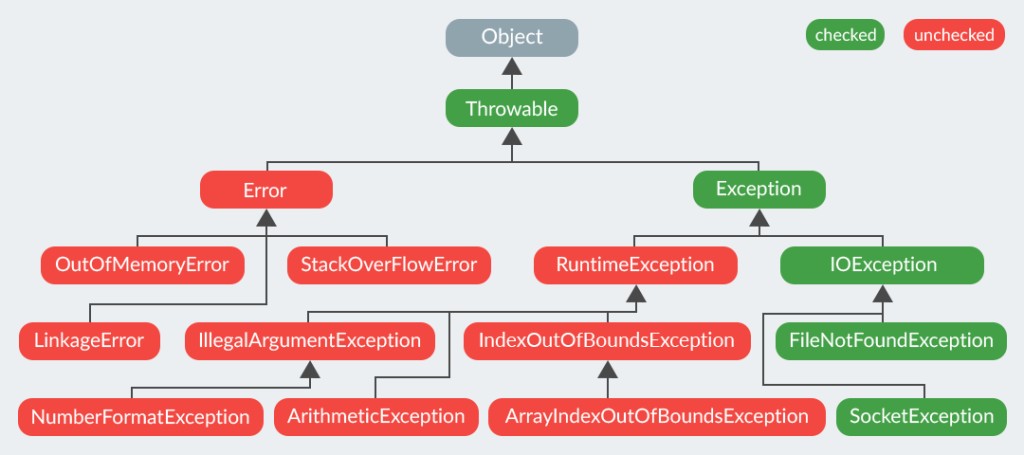
Exception
. What does “verifiable” mean? We partially mentioned this in the last lecture : “...the Java compiler knows about the most common exceptions, and knows in what situations they can occur.” For example, he knows that if a programmer reads data from a file in code, the situation could easily arise that the file does not exist. And there are a lot of such situations that he can predict in advance. Therefore, the compiler checks our code in advance for potential exceptions. If it finds them, it will not compile the code until we process them or forward them to the top. The second type of exception is “unchecked”. They come from the class RuntimeException
. How do they differ from those being tested? It would seem that there are also a bunch of different classes that come from RuntimeException
and describe specific runtime exceptions. The difference is that the compiler does not expect these errors. He seems to be saying: “At the time of writing the code, I did not find anything suspicious, but something went wrong while it was working. Apparently there are errors in the code!” And indeed it is. Unchecked exceptions are most often the result of programmer errors. And the compiler is clearly not able to provide for all possible incorrect situations that people can create with their own hands :) Therefore, it will not check the handling of such exceptions in our code. You've already encountered several unchecked exceptions:
ArithmeticException
occurs when divided by zeroArrayIndexOutOfBoundsException
Occurs when trying to access a cell outside the array.
How to throw your exception
Of course, the creators of Java are not able to provide for all exceptional situations that may arise in programs. There are too many programs in the world, and they are too different. But that's okay, because you can create your own exceptions if necessary. This is done very easily. All you have to do is create your own class. Its name must end with “Exception”. The compiler does not need this, but programmers reading your code will immediately understand that this is an exception class. In addition, you need to indicate that the class comes from the classException
. This is already necessary for the compiler and correct operation. For example, we have a class Dog - Dog
. We can walk the dog using the walk()
. But before that, we need to check whether our pet is wearing a collar, leash and muzzle. If any of these are missing, we'll throw our own exception DogIsNotReadyException
. Its code will look like this:
public class DogIsNotReadyException extends Exception {
public DogIsNotReadyException(String message) {
super(message);
}
}
To indicate that a class is an exception, you need to write extends Exception after the class name: this means that “the class derives from the class Exception.” In the constructor, we will simply call the class constructor Exception
with a line message
- it will display to the user a message from the system describing the error that has occurred. This is what it will look like in our class code:
public class Dog {
String name;
boolean isCollarPutOn;
boolean isLeashPutOn;
boolean isMuzzlePutOn;
public Dog(String name) {
this.name = name;
}
public static void main(String[] args) {
}
public void putCollar() {
System.out.println("The collar is on!");
this.isCollarPutOn = true;
}
public void putLeash() {
System.out.println("The leash is on!");
this.isLeashPutOn = true;
}
public void putMuzzle() {
System.out.println("The muzzle is on!");
this.isMuzzlePutOn = true;
}
public void walk() throws DogIsNotReadyException {
System.out.println("Let's go for a walk!");
if (isCollarPutOn && isLeashPutOn && isMuzzlePutOn) {
System.out.println("Hurrah, let's go for a walk!" + name + " I am glad!");
} else {
throw new DogIsNotReadyException("Dog " + name + "not ready for a walk! Check your gear!");
}
}
}
Now our method walk()
throws an exception DogIsNotReadyException
. This is done using the keyword throw
. As we said earlier, an exception is an object. Therefore, in our method, when an exceptional situation occurs - something is missing on the dog - we create a new class object DogIsNotReadyException
and throw it into the program using the word throw
. walk()
We add throws to the method signature DogIsNotReadyException
. In other words, the compiler is now aware that a method call walk()
may result in an exception. So when we call this somewhere in the program, the exception will need to be handled. Let's try to do this in the method main()
:
public static void main(String[] args) {
Dog dog = new Dog("Mukhtar");
dog.putCollar();
dog.putMuzzle();
dog.walk();//Unhandled exception: DogIsNotReadyException
}
Doesn't compile, exception not handled! Let's wrap our code in a block try-catch
to handle the exception:
public static void main(String[] args) {
Dog dog = new Dog("Mukhtar");
dog.putCollar();
dog.putMuzzle();
try {
dog.walk();
} catch (DogIsNotReadyException e) {
System.out.println(e.getMessage());
System.out.println("Checking equipment! Is the collar on?" + dog.isCollarPutOn + "\r\n Is the leash on?"
+ dog.isLeashPutOn + "\r\n Are you wearing a muzzle?" + dog.isMuzzlePutOn);
}
}
Now let's look at the console output:
Ошейник надет!
Намордник надет!
Собираемся на прогулку!
Собака Мухтар не готова к прогулке! Проверьте экипировку!
Проверяем снаряжение! Ошейник надет? true
Поводок надет? false
Намордник надет? true
Look how much more informative the console output has become! We see every step that happened in the program; We see where the error occurred and immediately notice what exactly our dog is missing :) This is how we create our own exceptions. As you can see, nothing complicated. And although the Java developers did not bother to add a special exception to their language for incorrectly equipped dogs, we corrected their oversight :)
GO TO FULL VERSION