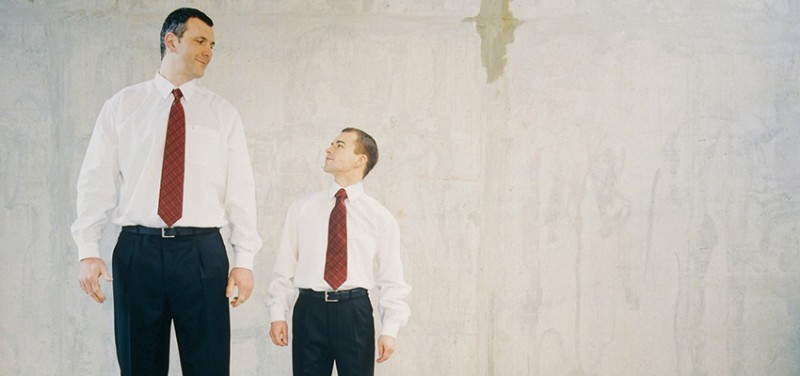
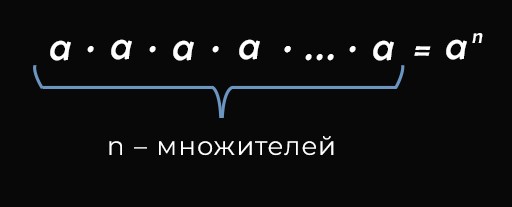
-
Math pow
The easiest way to solve this problem is to use the Math class. This is the solution you will use in most cases.
The Math class contains methods related to trigonometry, geometry, and other aspects of mathematics. In it, the methods are implemented as static, so you can immediately call through the class name Math without creating a class object.
How we will look like exponentiation:
public static int pow(int value, int powValue) { return (int) Math.pow(value, powValue); }
We had to use a type cast (int), since this method of the Math class returns a value of type double (the arguments are also double, but implicit type casting is used there).
And now - a bonus: additional options.
-
The value of the square of a number
Let's start with the most simple.
This is how the method for squaring is written:
public static int pow(int value){ return value*value; }
Call to main:
public static void main(String[] args) { System.out.println(Solution.pow(7)); }
That's all - nothing complicated and superfluous.
-
Number to the power
But the number squared is far from all we need. Most often, we will need a number in our work to a certain extent, so the following is a slightly more complicated version, but with a custom java pow value:
public static void main(String[] args) { System.out.println(Solution.pow(7, 4)); } public static int pow(int value, int powValue) { int result = 1; for (int i = 1; i <= powValue; i++) { result = result * value; } return result; }
The algorithm is very simple: we kind of set the starting point of result, and then multiply it by our value value as many times as the loop with powValue will run (powValue number of times)
-
recursion
The next way will be a little more exotic, but no less cool.
Recursion is a facility that allows a method to call itself. Java has such a mechanism, and such methods are called recursive methods, respectively.
Many, if not all, algorithmic problems can be solved recursively. This one will be no exception either, so let's take a look at how you can raise a number to a certain power in a recursive way:
public static int pow(int value, int powValue) { if (powValue == 1) { return value; } else { return value * pow(value, powValue - 1); } }
As you can see, we have two cases:
- The condition for exiting the recursion, or in other words, when our degree value reaches one, we will begin to be thrown back.
- The very mechanism of multiplying value by the result of calling the same method, but with powValue - 1.
Well, now it's time to look at more lazy ways, namely, out-of-the-box methods.
-
BigInteger
The main purpose of the BigInteger class is to store integers of arbitrary size, but at the same time it has various arithmetic methods that allow you to work with these huge (well, or not so) numbers.
You can read more about BigInteger in this article .
So what would exponentiation look like with BigInteger in Java?
public static int pow(int value, int powValue) { BigInteger a = new BigInteger(String.valueOf(value)); return a.pow(powValue).intValue(); }
Pretty simple and no frills, right?

GO TO FULL VERSION