
Libraries and standards
52. What is Hibernate? What is the difference between JPA and Hibernate?
I think to answer this question, we first need to understand what JPA is . JPA is a specification that describes the object-relational mapping of simple Java objects and provides an API for storing, retrieving, and manipulating such objects. That is, as we remember, relational databases (DBs) are presented in the form of many interconnected tables. And JPA is a widely accepted standard that describes how objects can interact with relational databases. As you can see, JPA is something abstract and intangible. It’s like the idea itself, the approach.
53. What is cascading? How is it used in Hibernate?
As I said earlier, in Hibernate communication is carried out through data objects called entities . These entities represent some specific tables in the database, and as you remember, in Java classes can contain references to other classes. These relationships are reflected in the database. In a database, as a rule, these are either foreign keys (for OneToOne, OneToMany, ManyToOne) or intermediate tables (for ManyToMany). You can read more about the relationship between entities in this article . When your entity has links to other related entities, annotations are placed above these links to indicate the type of connection: @OneToOne, @OneToMany, @ManyToOne, @ManyToMane, in whose parameters you can specify the value of the property - cascade - the type of cascade for this connection. JPA has specific methods for interacting with entities (persist, save, merge...) . Cascading types are precisely used to show how the associated data should behave when these methods are used on the target entity. So, what are the cascading strategies (types of cascading)? The JPA standard implies the use of six types of cascading:-
PERSIST - save operations will occur in cascade (for the save() and persist() methods ). That is, if we save an entity associated with other entities, they are also saved in the database (if they are not already there)
-
MERGE - update operations will occur in cascade (for the merge() method )
-
REMOVE - removal operations occur in cascade ( remove() method )
-
ALL - contains three cascade operations at once - PERSIST - MERGE - REMOVE
-
DETACH - related entities will not be managed by the session ( detach() method ). That is, when they change, there will be no automatic change in their data in the database - they are transferred from the persistence state to detached (an entity not managed by JPA)
-
REFRESH - every time an entity is updated with data from the database ( refresh() - updates detached objects), related entities are updated in the same way. For example, you somehow changed the data taken from the database and want to return its original values. In this case, this operation will be useful to you.
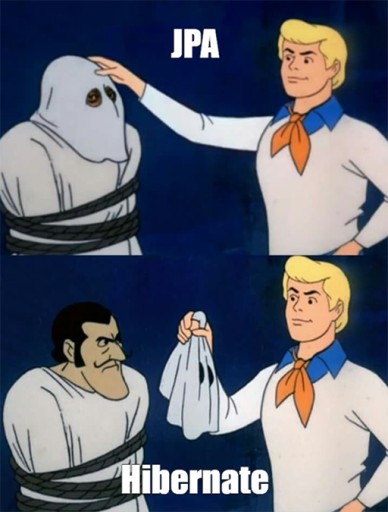
-
REPLICATE - Used when we have more than one data source and we want the data to be synchronized (Hibernate method - replicate). All entities must have identifiers (id) so that there are no problems with their generation (so that the same entity does not have different ids for different databases)
-
SAVE_UPDATE - cascade save/delete (for the Hibernate method - saveOrUpdate )
-
LOCK is the inverse operation to DETACHED : it transfers the detached entity back to the persistence state , i.e. entity will become tracked again by the current session
54. Can an Entity class be abstract?
In the JPA specification in paragraph 2.1 The Entity Class there is a line: “ Both abstract and concrete classes can be entities .” So the answer is yes, an abstract class can be an entity and can be annotated with @Entity.55. What is an entity manager? What is he responsible for?
First of all, I would like to note that EntityManager is one of the key components of JPA , which is used to interact entities with the database. In general, it calls the methods of interaction between the entity and the database (persist, merge, remove, detach)... But I would also note that this component, as a rule, is not one for the entire application: most often it is lightweight and is often removed and a new one is created using EntityManagerFactory . If we draw a parallel with JDBC , where EntityManagerFactory will be an analogue of DataSource , then EntityManager, in turn, will be an analogue of Connection . Earlier I mentioned a persistence entity , as an entity that is controlled by the current connection. So: this entity is managed precisely by the EntityManager , which is closely related to the current connection and the TransactionManager , which is responsible for opening/closing transactions. Further in the figure below you can see the life cycle of an entity:
56. What is the Assert class? Why use it?
I have not heard of such a class in JPA , so I will assume that this refers to the JUnit class of the library, which is used for unit testing of code. This library's class, Assert , is used to check the results of code execution ( assert is a statement that you have a certain state/data in a certain place). For example, you are testing a method that should create a cat. You run a method and get some result:Cat resultOfTest = createCat();
But you need to make sure it was created correctly, right? Therefore, you previously created a certain cat - expectedCat - manually with exactly the parameters that you expect from the cat obtained from the createCat() method . Next, you use the Assert class to verify the results:
Assert.assertEquals(resultOfTest, expectedCat);
If the cats are different, an AssertionError exception will be thrown , which tells us that the expected results do not converge. The Assert class has many different methods that cover many of the tasks of checking the expected results. Here are some of them:
-
assertTrue(<boolean>) - the expected value received as an argument must be true
-
assertFalse(<boolean>) - the expected value received as an argument should be false
-
assertNotEquals(<object1>, <object2>) - objects received as arguments must be different when compared using equals ( false )
-
assertThrows(<ClassNameOfException>.class, <exceptionObject>) - the second argument is expected to be an exception of the class specified by the first argument (i.e., as a rule, in place of the second argument, a method is called that should throw an exception of the required type)
String
57. Characterize String in Java
String is a standard class in Java, responsible for storing and manipulating string values (sequences of characters), is an immutable class ( I wrote about immutable earlier ), i.e. The data of objects of this class cannot be changed after creation. I would like to immediately note that the StringBuilder and StringBuffer classes are two virtually identical classes with the only difference being that one of them is intended for use in a multi-threaded environment (StringBuffer). These classes are analogous to String , but unlike it, they are mutable . That is, objects, once created, allow modification of the string they represent without creating a new object. Actually, the methods differ from the standard String methods and are aimed at meeting the needs of changing the string (it’s not for nothing that they’re called a builder). Read more about String , StringBuffer and StringBuilder in this article .58. What are the different ways to create a String object? Where is it created?
The most common way to create a string is to simply specify the value we need in double brackets:String str = "Hello World!";
You can also do this directly via new :
String str = new String("Hello World!");
You can create a string starting from an array of characters:
char[] charArr = {'H','e','l','l','o',' ', 'W','o','r','l','d','!'};
String str = new String(charArr);
As a result of the toString method running on some object:
String str = someObject.toString();
Like the result of any other method, it returns a string representation. For example:
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String str = reader.readLine();
As you understand, there can be very, very many ways to create a string. When a String object is created, it is stored in the string pool , which we will talk about in more detail in one of the questions below.
59. How to compare two strings in Java and how to sort them?
Java uses the double equals sign == to compare values . If we needed to compare some simple values like int , we would use it. But this method is not applicable for comparing full-fledged objects. In this case, it will only be a comparison of references - whether they point to the same object or not. That is, when comparing two objects with exactly the same values of internal fields, comparison through == will give the result false : despite the identical fields of the objects, the objects themselves occupy different memory cells. And objects of the String class , despite their deceptive simplicity, are still objects. And comparison via == is also not applicable for them (even despite the presence of a string pool). Here the standard method of the Object class comes into play - equals , which must be overridden in the class for it to work correctly (otherwise, by default it compares using == ). It is overridden in the String class , so we just take it and use it:String firstStr = "Hello World!";
String secondStr = "Hello World!";
boolean isEquals = firstStr.equals(secondStr);

TreeSet<String> sortedSet = new TreeSet<>();
sortedSet.add("B");
sortedSet.add("C");
sortedSet.add("A");
sortedSet.forEach(System.out::println);
Console output:
60. Give an algorithm for converting a string into a character. Write the appropriate code
As I said earlier, objects of the String class have a lot of different useful methods. One of these is toCharArray . This method converts a string to a character array:String str = "Hello world";
char[] charArr = str.toCharArray();
Next we have an array of characters that we can call by index:
char firstChar = charArr[0]; // H
61. How to convert a string to a byte array and back? Write the appropriate code
Similar to the toCharArray method , the String class has a getBytes method that returns a byte array of the string:String str = "Hello world";
byte[] byteArr = str.getBytes();
byte firstChar = byteArr[6]; // 119
Today's part of the analysis has come to its logical end. Thank you for your attention!
GO TO FULL VERSION