Hey everyone! Programming is full of pitfalls. And there is practically no topic in which you will not stumble and get bumps. This is especially true for beginners. The only way to reduce this is to study. In particular, this applies to detailed analyzes of the most basic topics. Today I continue to analyze 250+ questions from Java developer interviews, which cover basic topics well. I would like to note that the list also contains some non-standard questions that allow you to look at common topics from a different angle.
62. What is a string pool and why is it needed?
In memory in Java (Heap, which we will talk about later) there is an area - String pool , or string pool. It is designed to store string values. In other words, when you create a certain string, for example through double quotes:String str = "Hello world";
a check is made to see if the string pool has the given value. If so, the str variable is assigned a reference to that value in the pool. If it does not, a new value will be created in the pool, and a reference to it will be assigned to the str variable . Let's look at an example:
String firstStr = "Hello world";
String secondStr = "Hello world";
System.out.println(firstStr == secondStr);
true will be displayed on the screen . We remember that == compares references—meaning these two references refer to the same value from the string pool. This is done in order not to produce many identical objects of the String type in memory, because as we remember, String is an immutable class, and if we have many references to the same value, there is nothing wrong with that. It is now impossible for a situation in which changing a value in one place to change several other links at once. But nevertheless, if we create a string using new :
String str = new String("Hello world");
a separate object will be created in memory that will store this string value (and it does not matter whether we already have such a value in the string pool). As confirmation:
String firstStr = new String("Hello world");
String secondStr = "Hello world";
String thirdStr = new String("Hello world");
System.out.println(firstStr == secondStr);
System.out.println(firstStr == thirdStr);
We'll get two false values , which means we have three different values here that are being referenced. Actually, this is why it is recommended to create strings simply using double quotes. However, you can add (or get a reference to) values into a string pool when creating an object using new . To do this, we use the string class method - intern() . This method forcibly creates a value in the string pool, or gets a link to it if it is already stored there. Here's an example:
String firstStr = new String("Hello world").intern();
String secondStr = "Hello world";
String thirdStr = new String("Hello world").intern();
System.out.println(firstStr == secondStr);
System.out.println(firstStr == thirdStr);
System.out.println(secondStr == thirdStr);
As a result, we will receive three true values in the console , which means that all three variables refer to the same string.
63. What GOF patterns are used in the string pool?
The GOF pattern is clearly visible in the string pool - flyweight , otherwise called settler. If you see another template here, share it in the comments. Well, let's talk about the lightweight template. Lightweight is a structural design pattern in which an object that presents itself as a unique instance in different places in the program, in fact, is not so. Lightweight saves memory by sharing the shared state of objects among themselves, instead of storing the same data in each object. To understand the essence, let's look at the simplest example. Let's say we have an employee interface:public interface Employee {
void work();
}
And there are some implementations, for example, lawyer:
public class Lawyer implements Employee {
public Lawyer() {
System.out.println("Юрист взят в штат.");
}
@Override
public void work() {
System.out.println("Решение юридических вопросов...");
}
}
And the accountant:
public class Accountant implements Employee{
public Accountant() {
System.out.println("Бухгалтер взят в штат.");
}
@Override
public void work() {
System.out.println("Ведение бухгалтерского отчёта....");
}
}
The methods are very conditional: we just need to see that they are carried out. The same situation applies to the constructor. Thanks to the console output, we will see when new objects are created. We also have an employee department, whose task is to issue the requested employee, and if he is not there, hire him and issue in response to the request:
public class StaffDepartment {
private Map<String, Employee> currentEmployees = new HashMap<>();
public Employee receiveEmployee(String type) throws Exception {
Employee result;
if (currentEmployees.containsKey(type)) {
result = currentEmployees.get(type);
} else {
switch (type) {
case "Бухгалтер":
result = new Accountant();
currentEmployees.put(type, result);
break;
case "Юрист":
result = new Lawyer();
currentEmployees.put(type, result);
break;
default:
throw new Exception("Данный сотрудник в штате не предусмотрен!");
}
}
return result;
}
}
That is, the logic is simple: if there is a given unit, return it; if not, create it, place it in storage (something like a cache) and give it back. Now let's see how it all works:
public static void main(String[] args) throws Exception {
StaffDepartment staffDepartment = new StaffDepartment();
Employee empl1 = staffDepartment.receiveEmployee("Юрист");
empl1.work();
Employee empl2 = staffDepartment.receiveEmployee("Бухгалтер");
empl2.work();
Employee empl3 = staffDepartment.receiveEmployee("Юрист");
empl1.work();
Employee empl4 = staffDepartment.receiveEmployee("Бухгалтер");
empl2.work();
Employee empl5 = staffDepartment.receiveEmployee("Юрист");
empl1.work();
Employee empl6 = staffDepartment.receiveEmployee("Бухгалтер");
empl2.work();
Employee empl7 = staffDepartment.receiveEmployee("Юрист");
empl1.work();
Employee empl8 = staffDepartment.receiveEmployee("Бухгалтер");
empl2.work();
Employee empl9 = staffDepartment.receiveEmployee("Юрист");
empl1.work();
Employee empl10 = staffDepartment.receiveEmployee("Бухгалтер");
empl2.work();
}
And in the console, accordingly, there will be an output:
The lawyer has been hired. Solving legal issues... An accountant has been hired. Maintaining an accounting report.... Solving legal issues... Maintaining an accounting report.... Solving legal issues... Maintaining an accounting report.... Solving legal issues... Maintaining an accounting report.... Solving legal issues. .. Maintaining accounting reports...
As you can see, only two objects were created, which were reused many times. The example is very simple, but it clearly demonstrates how using this template can save our resources. Well, as you noticed, the logic of this pattern is very similar to the logic of the insurance pool. You can read more about the types of GOF patterns in this article .
64. How to split a string into parts? Please provide an example of the corresponding code
Obviously, this question is about the split method . The String class has two variations of this method:String split(String regex);
And
String split(String regex);
regex is a line separator - some regular expression that divides a string into an array of strings, for example:
String str = "Hello, world it's Amigo!";
String[] arr = str.split("\\s");
for (String s : arr) {
System.out.println(s);
}
The following will be output to the console:
Hello, world it's Amigo!
That is, our string value was split into an array of strings and the separator was a space (for separation, we could use a non-space regular expression "\\s" and just a string expression " " ). The second, overloaded method has an additional argument - limit . limit — the maximum allowed value of the resulting array. That is, when the string has already been split into the maximum allowable number of substrings, there will be no further splitting, and the last element will have a “remainder” of the possibly under-split string. Example:
String str = "Hello, world it's Amigo!";
String[] arr = str.split(" ", 2);
for (String s : arr) {
System.out.println(s);
}
Console output:
Hello, world it's Amigo!
As we can see, if not for the limit = 2 constraint , the last element of the array could be split into three substrings.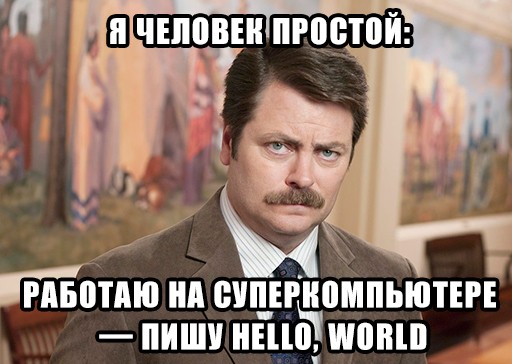
65. Why is a character array better than a string for storing a password?
There are several reasons to prefer an array to a string when storing a password: 1. String pool and string immutability. When using an array ( char[] ), we can explicitly erase the data after we're done with it. Also, we can rewrite the array as much as we want, and the valid password will not be anywhere in the system, even before garbage collection (it is enough to change a couple of cells to invalid values). At the same time, String is an immutable class. That is, if we want to change its value, we will get a new one, while the old one will remain in the string pool. If we want to remove the String value of a password, this can be a very difficult task, since we need the garbage collector to remove the value from the String pool , and there is a high probability that this String value will remain there for a long time. That is, in this situation, String is inferior to the char array in terms of data storage security. 2. If the String value is accidentally output to the console (or logs), the value itself will be displayed:String password = "password";
System.out.println("Пароль - " + password);
Console output:
Password
At the same time, if you accidentally output an array to the console:
char[] arr = new char[]{'p','a','s','s','w','o','r','d'};
System.out.println("Пароль - " + arr);
There will be incomprehensible gobbledygook in the console:
Password - [C@7f31245a
Actually not gobbledygook, but: [C is the class name is a char array , @ is a separator, followed by 7f31245a is a hexadecimal hashcode. 3. The official document, the Java Cryptography Architecture Guide, explicitly mentions storing passwords in char[] instead of String : “It would seem logical to collect and store a password in an object of type java.lang.String . However, there is a caveat here: String objects are immutable, i.e., there are no methods defined to allow the contents of a String object to be modified (overwritten) or nulled after use. This feature makes String objects unsuitable for storing sensitive information such as user passwords. Instead, you should always collect and store sensitive security information in a character array.”
Enum
66. Give a brief description of Enum in Java
Enum is an enumeration, a set of string constants united by a common type. Declared through the keyword - enum . Here is an example with enum - valid roles in a certain school:public enum Role {
STUDENT,
TEACHER,
DIRECTOR,
SECURITY_GUARD
}
The words written in capital letters are the same enumeration constants that are declared in a simplified way, without using the new operator . Using enumerations greatly simplifies life, as they help to avoid errors and confusion in naming (since there can only be a certain list of values). Personally, I find them very convenient when used in the logic design of Switch .
67. Can Enum implement interfaces?
Yes. After all, enumerations must represent more than just passive collections (such as roles). In Java, they can represent more complex objects with some functionality, so you may need to add additional functionality to them. This will also allow you to use the capabilities of polymorphism by substituting the enum value in places where the type of implemented interface is required.68. Can Enum extend a class?
No, it can't, because an enum is a default subclass of the generic class Enum <T> , where T represents the generic enum type. It is nothing but a common base class for all enum types of Java language. The conversion of enum to class is done by the Java compiler at compile time. This extension is not explicitly indicated in the code, but is always invisibly present.69. Is it possible to create an Enum without object instances?
As for me, the question is a little strange, or I didn’t fully understand it. I have two interpretations: 1. Can there be an enum without values - yes of course it would be something like an empty class - meaningless:public enum Role {
}
And calling:
var s = Role.values();
System.out.println(s);
We will receive in the console:
[Lflyweight.Role;@9f70c54
(empty array of Role values ) 2. Is it possible to create an enum without the new operator - yes, of course. As I said above, you do not need to use the new operator for enum values (enumerations) , since these are static values.
70. Can we override toString() method for Enum?
Yes, of course you can override the toString() method to define a specific way to display your enum when calling the toString method (when translating the enum into a regular string, for example, for output to the console or logs).public enum Role {
STUDENT,
TEACHER,
DIRECTOR,
SECURITY_GUARD;
@Override
public String toString() {
return "Выбрана роль - " + super.toString();
}
}
That's all for today, until the next part!
GO TO FULL VERSION