What is the difference between HashMap and Hashtable
Source: Medium Today you will learn the answer to one of the most popular Java interview questions: what is the difference between HashMap and Hashtable.
How to find and fix NullPointerException in Java
Source: Theflashreads After reading this article, you will be better able to find and fix a common error in Java code - NullPointerException.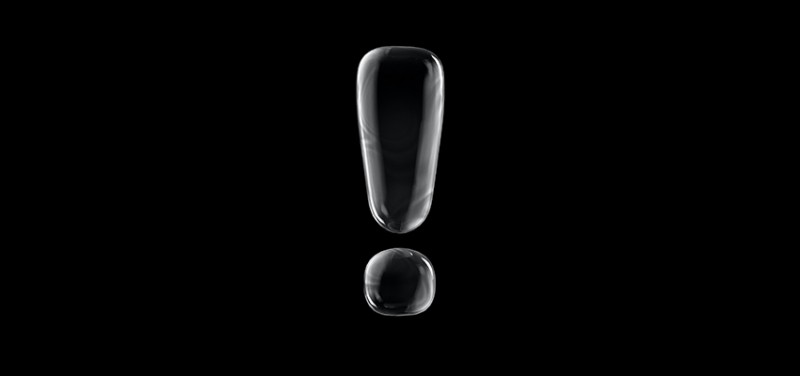
What is a NullPointerException?
java.lang.NullPointerException is a runtime exception in Java. It occurs when an attempt is made to access a variable that does not point to or refer to any object, that is, it is null. Because NullPointerException is a run-time exception, it does not need to be caught and handled explicitly in application code.Under what circumstances does a NullPointerException occur?
NullPointerException occurs when an attempt is made to access an uninitialized object. As already mentioned, this happens when the object reference goes nowhere and has a null value. Here are some of the most common NullPointerException exception scenarios :- Access properties of a null object.
- Throwing null from a method that throws an exception.
- Incorrect configuration for dependency injection frameworks, for example: (Spring).
- Passing null parameters to a method.
- Calling methods on a null object.
- Using synchronized on a null object.
- Access the index element (for example, in an array) of a null object.
Example NullPointerException
In this example, we are trying to call the printLength method , which takes a string as a parameter and prints its length. If the value of the string that is passed as a parameter is null, a java.lang.NullPointerException will be thrown .public class NullPointerExceptionExample {
private static void printLength(String str) {
System.out.println(str.length());
}
public static void main(String args[]) {
String nullString = null;
printLength(nullString);
}
}
Exception in thread "main" java.lang.NullPointerException
at NullPointerExceptionExample.printLength(NullPointerExceptionExample.java:3)
at NullPointerExceptionExample.main(NullPointerExceptionExample.java:8)
How to avoid throwing NullPointerException
NullPointerException can be avoided by using the following checks and preventative methods:-
Ensure that an object is properly initialized by adding a null check before accessing its methods or properties.
-
Use Apache Commons StringUtils for string operations: for example, StringUtils.isNotEmpty() to check whether a string is empty before using it further.
import org.apache.commons.lang3.StringUtils; public class NullPointerExceptionExample { private static void printLength(String str) { if (StringUtils.isNotEmpty(str)) { System.out.println(str.length()); } else { System.out.println("This time there is no NullPointerException"); } } public static void main(String args[]) { String nullString = null; printLength(nullString); } }
-
Use primitives rather than objects whenever possible, since they cannot have null references. For example, use int instead of Integer and boolean instead of Boolean .
-
Make sure you double check your code. This will reduce the likelihood of a NullPointerException being thrown .
GO TO FULL VERSION