- Multidimensional arrays, general syntax
- 2D arrays
- Declaring, creating and initializing two-dimensional arrays
- Displaying a two-dimensional array on the screen
- Fast output of a two-dimensional array
- "Lengths" of a two-dimensional array
- 2D Array Example: Checkerboard
- 2D Array Example: Matrix Multiplication
- Non-rectangular 2D arrays
- 3D arrays
- Multidimensional arrays in the real work of a Java programmer
- Interesting tasks for two-dimensional and three-dimensional arrays

What is a one-dimensional Java array?
An array is an ordered set of elements of the same type, primitive or reference. General information about arrays (mostly one-dimensional) can be found in the article “ Arrays in Java ” and in the course CodeGym . This article focuses on arrays whose elements are other arrays. Such arrays are called multidimensional. An array whose elements are other arrays, that is, an array of arrays, is called a two-dimensional array. Not all languages have multidimensional arrays like this, but Java does.Java Multidimensional Arrays, General Syntax
In a generalized form, multidimensional arrays in Java look like this:Data_type[dimension1][dimension2][]..[dimensionN] array_name = new data_type[size1][size2]….[sizeN];
Where Data_type
is the type of the elements in the array. Can be primitive or reference (class). The number of pairs of brackets with dimension
inside is the dimension of the array (in our case, N
). array_name
- name of the array size1...sizN
- the number of elements in each of the dimensions of the array. Declaring multidimensional arrays:
int[][] twoDimArray; //two-dimensional array
String[][][] threeDimArray; //three-dimensional array
double[][][][][] fiveDimArray; // five-dimensional array
Perhaps all this looks very abstract, so now let's move on to specific manifestations of multidimensional arrays - two-dimensional and three-dimensional. The fact is that Java developers sometimes use two-dimensional arrays, much less often three-dimensional ones, and even larger arrays are extremely rare. With a high degree of probability, you will not encounter them.
Multidimensional arrays in CodeGym course On CodeGym, "regular" arrays are started at level 7 of the Java Syntax quest , and further along the course they are encountered repeatedly. Sometimes during the course there are problems on two-dimensional arrays (or those that can be solved with their help). Also, two-dimensional arrays are used in the game engine of the special section “ CodeGym Games ”. If you haven't been there yet, drop by and create a couple of games. Detailed instructions are attached to the conditions, and this will serve as an excellent training in programming skills. A three-dimensional array can be found in the game Space Invaders. Through it, a set of frames for animation is set (and each of these frames is a two-dimensional array). If you've already completed the JavaSyntax quest or just feel comfortable with Java programming, try writing your own version of this classic game. |
What is a two dimensional Java array?
A two-dimensional array in Java is an array of arrays, that is, in each of its cells there is a link to an array. But it is much easier to represent it as a table, which has a given number of rows (the first dimension) and the number of columns (the second dimension). A two-dimensional array in which all rows have the same number of elements is called a rectangular array.Declaring, creating and initializing two-dimensional arrays
The procedure for declaring and creating a two-dimensional array is almost the same as in the case of a one-dimensional one:int[][] twoDimArray = new int[3][4];
This array has 3 rows and 4 columns. The size of a rectangular two-dimensional array (they may not be rectangular, more on that below), that is, the total number of elements can be determined by multiplying the number of rows by the number of columns. Now it is initialized (filled) with default values. That is, zeros. Let's fill it with the values we need.
twoDimArray[0][0] = 5;//write the value 5 into the cell at the intersection of the zero row and zero column
twoDimArray[0][1] = 7; //write the value 7 into the cell at the intersection of the zero row and the first column
twoDimArray[0][2] = 3;
twoDimArray[0][3] = 17;
twoDimArray[1][0] = 7;
twoDimArray[1][1] = 0;
twoDimArray[1][2] = 1;
twoDimArray[1][3] = 12;
twoDimArray[2][0] = 8;
twoDimArray[2][1] = 1;
twoDimArray[2][2] = 2;
twoDimArray[2][3] = 3;
As in the case of one-dimensional arrays, you can carry out the initialization procedure faster:
int [][] twoDimArray = {{5,7,3,17}, {7,0,1,12}, {8,1,2,3}};
In both cases, we get a two-dimensional array with three rows and four columns, filled with integers. 
Displaying a two-dimensional array on the screen
This operation is most logical to do like this: first, we display the zero string element by element, then the second one, and so on. Most often in Java, the output of a two-dimensional array is implemented using two nested loops.int [][] twoDimArray = {{5,7,3,17}, {7,0,1,12}, {8,1,2,3}};//declared an array and filled it with elements
for (int i = 0; i < 3; i++) { //go through the lines
for (int j = 0; j < 4; j++) {//go through the columns
System.out.print(" " + twoDimArray[i][j] + " "); //output element
}
System.out.println();// line wrap for the visual preservation of the tabular form
}
Fast output of a two-dimensional array
The shortest way to display a list of elements of a two-dimensional array is to usedeepToString
the Arrays
. Example:
int[][] myArray = {{18,28,18},{28,45,90},{45,3,14}};
System.out.printLn(Arrays.deepToString(myArray));
The result of the program is the following output: [[18, 28, 18], [28, 45, 90], [45, 3, 14]]
"Lengths" of a two-dimensional array
To get the length of a one-dimensional array (that is, the number of elements in it), you can use the variablelength
. That is, if we define an array int a[] = {1,2,3}
, then the operation a.length
returns 3. But what if we apply the same procedure to our two-dimensional array?
int [][] twoDimArray = {{5,7,3,17}, {7,0,1,12}, {8,1,2,3}};
System.out.println(twoDimArray.length);
Output: 3 So this operation outputs the number of rows in the array. How to get the number of columns? If we are dealing with rectangular two-dimensional arrays (that is, those with all rows of the same length), then we can apply the operation twoDimArray[0].length
or instead of a zero element (in fact, a zero row) - any other existing one. We can do this because in Java a two-dimensional array is an array of arrays, and element zero twoDimArray[0]
is an array of length 4. You can check this yourself.
2D Array Example: Checkerboard
Two-dimensional arrays can be used to create any finite two-dimensional field, for example, in games, and in particular in chess. A chessboard is easy to represent as a two-dimensional array. You can “attach” graphics to this, but for now, let's set up a chess field using symbols and output it to the console.
W
(white), and the black cell with the letter B
(black).
//set the chessboard as a two-dimensional array
String [][] chessBoard = new String[8][8];
for (int i = 0; i< chessBoard.length; i++) {
for (int j = 0; j < chessBoard[0].length; j++) {
if ((i + j) % 2 == 0) chessBoard[i][j] = "W";
else chessBoard[i][j] = "B";
}
}
The output of the program is: WBWBWBWBBWBWBWBWWBWBW BWBBWBWBWBWWBWBWBWBBW BWBWBWWBWBWBWBBWBWBWB W Everything is like on a real chessboard, you can check it. 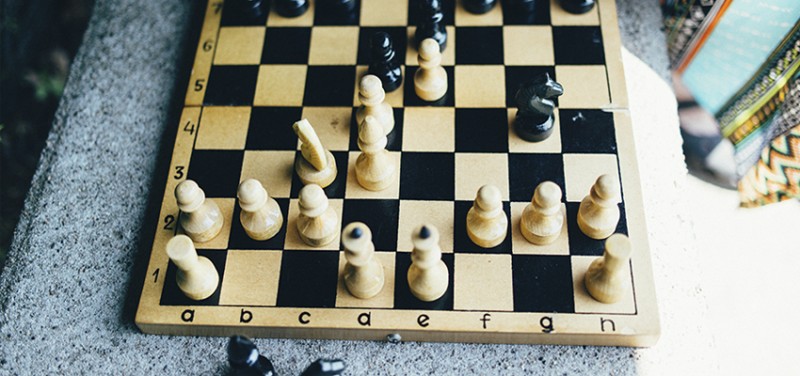
chessBoard[7][0]
. Let's compare each pair of indices of a two-dimensional array with their “chess” equivalent. To do this, we use two lines - " abcdefgh
" and " 87654321
" (in reverse order - for simplicity, so that the chess 8 corresponds to the zero column).
public static String chessBoardCoord(int a, int b) {
String letters = "abcdefgh";
String numbers = "87654321";
if ((a > 7)|| (b>7)) return null; //if the number is outside the board, return the default value - null
else return (Character.toString(letters.charAt(a)) + numbers.charAt(b)); /*charAt - a method with which we extract from the string the element under the passed number, here - under the numbers a and b. Character.toString - a method that converts the received character into a string */
}
Now we will display in each cell not only its color, but also its number, using the methodchessBoardCoord
String [][] chessBoard = new String[8][8];
for (int i = 0; i < chessBoard.length; i++) {
for (int j = 0; j < chessBoard[0].length; j++) {
if ((i + j) % 2 == 0) chessBoard[i][j] = "W" + chessBoardCoord(j,i);
else chessBoard[i][j] = "B"+ chessBoardCoord(j,i);
}
}
for (int i = 0; i < chessBoard.length; i++) {
for (int j = 0; j < chessBoard[0].length; j++) {
System.out.print(" " + chessBoard[i][j] + " ");
}
System.out.println();
}
Output: Wa8 Bb8 Wc8 Bd8 We8 Bf8 Wg8 Bh8 Ba7 Wb7 Bc7 Wd7 Be7 Wf7 Bg7 Wh7 Wa6 Bb6 Wc6 Bd6 We6 Bf6 Wg6 Bh6 Ba5 Wb5 Bc5 Wd5 Be5 Wf5 Bg5 Wh5 Wa4 Bb4 Wc4 Bd4 We4 Bf4 Wg4 Bh4 Ba3 Wb3 Bc3 Wd3 Be3 Wf3 Bg3 Wh3 Wa2 Bb2 Wc2 Bd2 We2 Bf2 Wg2 Bh2 Ba1 Wb1 Bc1 Wd1 Be1 Wf1 Bg1 Wh1 Where We2
means the white cell with number e2.
2D Array Example: Matrix Multiplication
Attention!This example requires basic knowledge about matrices. Here, very little will be told about them, and this information is intended for those who have studied, but somewhat forgotten, matrix arithmetic. However, this knowledge can be gleaned from open sources, in particular from the Wikipedia article . This is a good example of using two-dimensional arrays, but we can move on without it. So if it seems incomprehensible to you now from the point of view of mathematics, and you do not want to delve into it too much, feel free to skip the example. If you've been learning the basics of linear algebra, you may have recognized rectangular arrays as rectangular matrices.

a[l][m]
and b[m][n]
. As a result of their multiplication, we should get a matrix c[l][n]
. To get the element c[0][0]
of the product matrix, you need the zero element of the zero row of the first matrixa[0][0]
multiply by the zero element of the second matrix, then multiply the first element of the first row of the first matrix by the first element of the first column of the second matrix, and so on, after which all the resulting products are added.
a[0][0]*b[0][0] + a[0][1]*b[1][0] + … + a[0][m-1]*b[m-1][0]
To obtain the second element of the first row of the result matrix, we perform the same procedure with the second row
a[1][0]*b[0][0] + a[1][1]*b[0][1] + … + a[0][m-1]*b[m-1][0]
And so on until the end of the line. Then we go to the next line and repeat the procedure until we run out of lines. That is, we multiply the rows of the first matrix with the columns of the second matrix. Below is the code for matrix multiplication. You can supplement it with a check for compliance with the above condition on the number of rows and columns.
//declaring two matrices
int [][] twoDimArray1 = {{1,0,0,0},{0,1,0,0},{0,0,0,0}};
int[][] twoDimArray2 = {{1,2,3},{1,1,1},{0,0,0},{2,1,0}};
//matrix multiplication process
int[][]twoDimArray3 = new int [twoDimArray1.length][twoDimArray2[0].length];
for (int i=0; i<twoDimArray3[0].length; i++)
for (int j=0; j<twoDimArray3.length; j++)
for (k=0; k<twoDimArray1[0].length; k++)
twoDimArray3[i][j] = twoDimArray3[i][j] + twoDimArray1[i][k] * twoDimArray2[k][j];
//output on display
for (int i = 0; i < twoDimArray3.length; i++) {
for (int j = 0; j < twoDimArray3[0].length; j++) {
System.out.print(" " + twoDimArray3[i][j] + " ");
}
System.out.println();
}
The program outputs the following result: 1 2 3 1 1 1 0 0 0
Non-rectangular 2D arrays
Since two-dimensional arrays are arrays of arrays in Java, each of the inner arrays can be of different lengths. When creating an array, we can specify only the number of rows and not specify the number of columns (that is, in fact, the length of these same rows). Consider an example.//declaring and creating an array, specifying only the number of rows
int [][] twoDimArray = new int[5][];
//initialize the array, filling it with arrays of different lengths
twoDimArray[0] = new int[]{1, 2, 3, 4, 5};
twoDimArray[1] = new int[]{1,2,3,4};
twoDimArray[2] = new int[]{1,2,3};
twoDimArray[3] = new int[]{1,2};
twoDimArray[4] = new int[]{1};
//display the resulting non-rectangular two-dimensional array on the screen
for (int i = 0; i < twoDimArray.length; i++) {
for (int j = 0; j < twoDimArray[i].length; j++) {
System.out.print(" " + twoDimArray[i][j] + " ");
}
System.out.println();
}
The output of the program is: 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1 Thus, the zeroth line of our array contains the array {1,2,3,4,5}
, and the fourth one contains the array {1}
.
3D arrays in Java
Following the common sense and logic of the Java language, a three-dimensional array can be called "an array of arrays of arrays" or "an array of which each element is a two-dimensional array". Moreover, these two-dimensional arrays can be different. Example:// create a three-dimensional array consisting of two two-dimensional arrays
int[][][] threeDimArr = new int[2][][];
//create the first 2D array of a 5x2 3D array
threeDimArr[0] = new int[5][2];
//create a second 2D array of a 1x1 3D array
threeDimArr[1] = new int[1][1];
But more often in practice there are three-dimensional arrays in which all three values are determined at once, an analogue of rectangular two-dimensional arrays. 
boolean
with the value false if the place is free and true if the place is occupied.
//set a boolean three-dimensional array. This car park has 3 floors, each of which can accommodate 2x5 = 10 cars. By default, all cells are empty (false)
boolean[][][] parkingLot = new boolean[3][2][5];
//two cars arrived and parked on the ground floor in cell [1][0] and [1][3]
parkingLot[0][1][0] = true;
parkingLot[0][1][3] = true;
//Output the array to the console
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 2; j++) {
for (int k = 0; k < 5; k++) {
System.out.print("arr[" + i + "][" + j + "][" + k + "] = " + parkingLot[i][j][k] + "\t");
}
System.out.println();
}
}
Multidimensional arrays in the real work of a Java programmer In reality, most Java developers don't encounter multidimensional arrays very often. However, there are a number of tasks for which this data structure is very well suited.
|
Interesting tasks for two-dimensional and three-dimensional arrays
You know enough about multidimensional arrays in Java and, if you feel strong enough, you can try to solve one of the problems below. They are difficult but interesting. Tic-tac-toe. Set the field 3x3, create two players who move alternately. Initially, the field is empty, and in each of the empty fields the first player can put a cross, and the second zero. The winner is the one who first collects three crosses or three zeros located in one row, one column or diagonally.
What else to read |
---|
- On a black square, the ant must turn 90° to the left, change the color of its cell to white, then step forward to the next cell.
- On a white cell, the ant turns 90° to the right and changes the color of its cell to black, then steps forward to the next cell.
n
given the initial position of the ant. The field can be filled randomly with zeros and ones (or denoted by the letters W
and B
, as we did in the chessboard example). We also need two more parameters - the horizontal and vertical position of the ant, as well as its direction at this step (north, south, west, east), while by default the ant faces north. You can try to model the Rubik's Cube using three-dimensional arrays. The standard Rubik's Cube has 6 faces, and each of them is a three-dimensional array of colored squares Color[][][] rubik = new Color[6][3][3]
. However, the implementation of the Rubik's Cube is not a trivial task.
Useful materials about arrays
Arrays (mostly one-dimensional, since they are much more often used in practice) are devoted to many articles on CodeGym. Pay attention to them.- Arrays in Java - about arrays for beginners with examples
- Something about arrays - a good detailed article about arrays
- The Arrays class and its use - the article describes some of the class methods
Array
- Arrays is the first CodeGym lesson on arrays.
- Return a zero-length array, not null - Efficient Programming author Joshua Bloch talks about the best way to return empty arrays
GO TO FULL VERSION