
- Questions that intersect with this series of articles , I will skip so as not to duplicate information once again. I recommend reading these materials, as they contain the most frequent (popular) Java Core interview questions.
- Questions on DOU are presented in Ukrainian, but I will have everything here in Russian.
- The answers could have been written in more detail, but I won’t, because then the answer to each question can drag on an entire article. Yes, and so in detail you will not be asked in any social security.
11. Name all the methods of the Object class
The Object class has 11 methods:- Class<?> getClass() - getting the class of the current object;
- int hashCode() - getting the hash code of the current object;
- boolean equals(Object obj) - comparison of the current object with another;
- Object clone() - creating and returning a copy of the current object;
- String toString() - getting the string representation of the object;
- void notify() - awakening one thread waiting on the monitor of a given object (thread selection is random);
- void notifyAll () - awakening all threads waiting on the monitor of this object;
- void wait() - switches the current thread to standby mode (freezes it) on the current monitor, works only in a synchronized block until some notify or notifyAll wakes up the thread;
- void wait(long timeout) - also freezes the current thread on the current monitor (on the current synchronized), but with a timer to exit this state (well, or again: until notify or notifyAll wakes up);
- void wait(long timeout, int nanos) - a method similar to the one described above, but with more accurate freeze exit timers;
- void finalize() - This method is called (finally) before this object is removed by the garbage collector. It is used to clean up occupied resources.
12. What is the difference between try-with-resources and try-catch-finally when dealing with resources?
Typically, when using try-catch-finally, a final block was used to close resources. Java 7 introduced a new kind of try-with-resources statement , similar to try-catch-finally for freeing resources, but more compact and readable. Let's remember what try-catch-finally looks like :String text = "some text......";
BufferedWriter bufferedWriter = null;
try {
bufferedWriter = new BufferedWriter(new FileWriter("someFileName"));
bufferedWriter.write(text);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
bufferedWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
Now let's rewrite this code, but using try-with-resources :
String text = "some text......";
try(BufferedWriter bufferedWriter =new BufferedWriter(new FileWriter("someFileName"))) {
bufferedWriter.write(text);
} catch (IOException e) {
e.printStackTrace();
}
Somehow it became easier, don't you think? In addition to simplification, there are a couple more points:
-
In try-with-resources , resources declared within parentheses (which will be closed) must implement the AutoCloseable interface and its only method, close() .
The close method is executed in an implicit finally block , otherwise how will the program understand how exactly to close the resource?
But, most likely, you will rarely write your resource implementations and their closing method.
-
Block execution sequence:
- try block .
- Implicit finally .
- The catch block that catches the exceptions in the previous steps.
- Explicit finally .
As a rule, exceptions that fell below the list interrupt those that fell above.
13. What are bitwise operations?
Bitwise operations are operations on bitstrings, which include logical operations and bitwise shifts. Boolean operations:-
bitwise AND - compares bit values, and along the way, any bit set to 0 (false) sets the corresponding bit in the result to 0. That is, if both compared values had a bit 1 (true), the resulting one will also be 1.
Denoted as - AND , &
Example: 10111101 & 01100111 = 00100101
-
bitwise OR is the inverse of the previous one. Any bit set to 1 sets the same bit in the result as 1. And accordingly, if both compared values had a bit of 0, the resulting one will also be 0.
Denoted as - OR , |
Example: 10100101 | 01100011 = 11100111
-
bitwise NOT - applied to a single value, flips (inverts) the bits. That is, those bits that were 1 will become 0; and those that were 0 will become 1.
Denoted as - NOT , ~
Example: ~10100101 = 01011010
bitwise XOR - compares bit values, and if both values have a bit equal to 1, then the result will be 0, and if both values have a bit of 0, the result will be 0. That is, for the result to be equal to 1, you need only one of the bits was equal to 1, and the second is equal to 0. Denoted as - XOR , ^
Example: 10100101^01100011 = 11000110
- 01100011 >> 4 = 00000110
- 01100011 << 3 = 00011000


14. What are the standard immutable class objects in Java?
Immutable is an object that does not allow its original parameters to be changed. Perhaps it has methods that return new objects of that type, with the parameters you wanted to change. Some standard immutable objects:- By far the most famous immutable object in Java is String;
- instances of wrapper classes that wrap standard types: Boolean, Character, Byte, Short, Integer, Long, Double, Float;
- objects that are usually used for especially LARGE numbers - BigInteger and BigDecimal;
- an object that is a unit in stack traces (for example, in an exception stack trace) StackTraceElement;
- an object of class File - can change files, but at the same time it itself is unchanged;
- UUID - which is often used as a unique element id;
- all class objects of the java.time package;
- Locale - used to define a geographic, political, or cultural region.
15. What are the advantages of an immutable object over regular objects?
- Such objects are safe when used in a multithreaded environment . By using them, you don't have to worry about data being lost due to thread race conditions. In contrast to working with ordinary objects: in this case, you will have to think very carefully and work out the mechanisms for using the object in a parallel environment.
- Immutable objects are good keys in a map, because if you use a mutable object and then the object changes its state, there can be confusion when using a HashMap: the object will still be present, and if you use containsKey() , then it may not be found .
- Immutable objects are great for storing immutable (constant) data that should never be changed while the program is running.
- “Atomic with respect to failure” - if an immutable object throws an exception, then it still will not remain in an undesirable (broken) state.
- These classes are easy to test.
- No additional mechanisms such as copy constructor and clone implementation are needed.
Questions about OOP

16. What are the advantages of OOP in general and in comparison with procedural programming?
So, the advantages of OOP:- Complex applications are easier to write than procedural programming, since everything is broken down into small modules - objects that interact with each other - and as a result, programming comes down to relationships between objects.
- Applications written with OOP are much easier to modify (as long as the design concepts are followed).
- Since the data and operations on them form a single entity, they are not smeared throughout the application (which is often the case with procedural programming).
- Information encapsulation protects the most critical data to work from the user.
- It is possible to reuse the same code with different data, because classes allow you to create many objects, each of which has its own attribute values.
- Inheritance and polymorphism also allow you to reuse and extend existing code (instead of duplicating similar functionality).
- Easier application extensibility than procedural approach.
- The OOP approach makes it possible to abstract away from implementation details.
17. Tell us what are the disadvantages of OOP
Unfortunately, they also exist:- OOP requires a lot of theoretical knowledge that you need to master before you can write anything.
- OOP ideas are not so easy to understand and apply in practice (you need to be a bit of a philosopher at heart).
- When using OOP, the performance of the software operation is slightly reduced due to the more complex organization of the system.
- The OOP approach requires more memory, since everything consists of classes, interfaces, methods, which take up much more memory than ordinary variables.
- The time spent on the initial analysis is greater than during the procedural one.
18. What is static and dynamic polymorphism
Polymorphism allows objects to behave differently for the same class or interface. There are two kinds of polymorphism, which are also known as early and late binding . Static polymorphism, or earlier binding:- occurs at compile time (early in the life cycle of a program);
- decides which method to execute at compile time;
- method overloading is an example of static polymorphism;
- early binding includes private, static and terminal methods;
- inheritance does not participate in early binding;
- static polymorphism involves not specific objects, but information about the class, the type of which is presented to the left of the variable name.
- occurs at runtime (while the program is running);
- dynamic polymorphism decides which particular implementation a method will have at run time;
- method overriding is an example of dynamic polymorphism;
- late binding is the assignment of a particular object, a reference of its type, or its superclass;
- inheritance is related to dynamic polymorphism.
19. Define the principle of abstraction in OOP
Abstraction in OOP is a way to isolate a set of meaningful characteristics of an object by excluding non-significant details. That is, when designing a program with an OOP approach, you focus on models in general, without going into the details of their implementation. Interfaces are responsible for abstraction in Java.. For example, you have a machine and this will be an interface. And various interactions with it - for example, start the engine, use the gearbox - these are functions that we use without going into implementation details. Because when you're driving, you don't think exactly how the gearbox does its job, or how the key starts the engine, or exactly how the steering wheel turns the wheels. And even if the implementation of one of this functionality is replaced (for example, the engine), you may not notice it. It doesn't matter to you: you don't go into implementation details. It is important for you that the action is performed. Actually, this is an abstraction from implementation details. At this point, we will stop today: to be continued!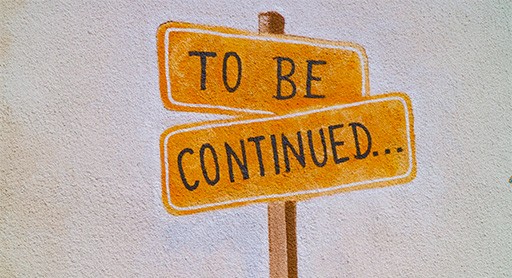

GO TO FULL VERSION