71. What happens if we don't override the toString() method for Enum?
Let's say we have the following enum :public enum Role {
STUDENT,
TEACHER,
DIRECTOR,
SECURITY_GUARD;
}
Let's display the student in the console by calling toString() on him :
System.out.println(Role.STUDENT.toString());
Result in the console:
72. Is it possible to specify a constructor inside an Enum?
Yes, sure. It is through the constructor that the values of the internal enum variables are set. As an example, let's add two fields to the previous enum - ageFrom and ageTo - to indicate the age range for each role:public enum Role {
STUDENT(5,18),
TEACHER(20,60),
DIRECTOR(40,70),
SECURITY_GUARD(18,50);
int ageFrom;
int ageTo;
Role(int ageFrom, int ageTo) {
this.ageFrom = ageFrom;
this.ageTo = ageTo;
}
}
73. What is the difference between == and equals()?
This is one of the most common Java developer interview questions. Let's start with the fact that when we compare simple values ( int , char , double ...), we do it using == , since variables contain specific values and we can compare them. And primitive variables are not full-fledged objects - they do not inherit from Object and do not have an equals() method . When we talk about comparing variables that refer to objects, == will only compare the value of the references - whether they refer to the same object or not. And even if one object is identical to another, comparison via == will give a negative result ( false ), because this is a different object. As you understand, the equals() method is used to compare reference variables . This is one of the standard methods of the Object class , which is needed for a complete comparison of objects. But it’s worth clarifying right away: for this method to work correctly, it needs to be redefined by writing exactly how objects of this class should be compared. Unless you override the method, by default it will compare objects by == . In IntelliJ IDEA , you can override it automatically (using IDEA tools) -> alt + insert , in the window that appears, select equals() and hashCode() -> select which class fields should participate -> and voila, automatic implementation of the methods is completed. Here is an example of what an automatically generated equals method would look like for a simple Cat class with two fields - int age and String name :@Override
public boolean equals(final Object o) {
if (this == o) return true;
if (o == null || this.getClass() != o.getClass()) return false;
final Cat cat = (Cat) o;
return this.age == cat.age &&
Objects.equals(this.name, cat.name);
}
If we talk about the difference between == and equals for enums , there isn’t much of it. 


74. What does the ordinal() method do in Enum?
When calling the int ordinal() method on an enum element , we will get the ordinal number from zero of this value in the general series of enumerations. Let's use this method on one element from the previous enum discussed - Role :System.out.println(Role.DIRECTOR.ordinal());
Accordingly, the console will display:
75. Is it possible to use Enum with TreeSet or TreeMap in Java?
The use of enum types in TreeSet and TreeMap is acceptable. And we can write:TreeSet<Role> treeSet = new TreeSet<>();
treeSet.add(Role.SECURITY_GUARD);
treeSet.add(Role.DIRECTOR);
treeSet.add(Role.TEACHER);
treeSet.add(Role.STUDENT);
treeSet.forEach(System.out::println);
And the console will display:

76. How are the ordinal() and compareTo() methods related in Enum?
As stated earlier, ordinal() returns the ordinal number of a value in a general enumeration list. Also, in the analysis of the previous question, you saw that the elements of enumerations, once, for example, in a TreeSet (sorted set) take the order in which they are declared in enum . And as we know, TreeSet and TreeMap sort elements by calling their compareTo() method of the Comparable interface . From this we can assume that the Enum class implements the Comparable interface , implementing it in the compareTo() method , within which ordinal() is used to set the sort order. Having entered the Enum class , we see confirmation of this:


77. Write an example EnumM
In the questions discussed above, I already gave examples of enums and I don’t see the point in duplicating the code (for example, question number 72 about the constructor in enum).78. Is it possible to use Enum in a switch case?
It is possible and necessary! Looking back at my practice, I note that one of the most common places to use enum is logical constructs like switch . In this case, you can provide all possible variations of case , and after writing the logic for all enum values - and using the default operator may not even be necessary! After all, if you use a String or a numeric value, for example, of type int , you may receive an unexpected value, which in turn is impossible using an enum . What would a switch look like for the example discussed earlier:public void doSomething(Role role) {
switch (role) {
case STUDENT:
// некая логика для STUDENT
break;
case TEACHER:
// некая логика для TEACHER
break;
case DIRECTOR:
// некая логика для DIRECTOR
break;
case SECURITY_GUARD:
// некая логика для SECURITY_GUARD
break;
}
}
79. How to get all the available values in an Enum instance?
If you need to get all instances of an enumeration, there is a values() method that returns an array of all available values of a particular enum in natural order (in the order they were specified in the enum ). Example:Role[] roles = Role.values();
for (Role role : roles) {
System.out.println(role);
}
The console will display the following output:
Stream API
80.What is Stream in Java?
Java Stream is a relatively new way of interacting with a data stream, which in turn allows you to more conveniently and compactly process large data, as well as parallelize data processing between a certain number of threads, which can give a performance boost in the using functionality. This topic cannot be discussed more deeply in a nutshell, so I will leave here a link to an article that can help you dive into this topic.
81. What are the main properties of transactions?
The topic is called Stream API, but the question is about the transaction. Hmm... First, let's figure out what a transaction is. A transaction is a group of sequential database operations that represents a logical unit of working with data. A transaction can be completed either entirely and successfully, maintaining data integrity and independently of other transactions running in parallel, or it can not be completed at all, in which case it has no effect. So, transactions have four main properties, which are called ACID for short . Let's look at how each letter of this abbreviation stands for: A - Atomicity - atomicity - this property guarantees that no transaction will be partially recorded in the system. Either all of its sub-operations will be performed, or none will be performed ( all or nothing ). C - Consistency - consistency is a property that ensures that each successful transaction records only valid results. That is, this is a guarantee that in the event of a successful transaction, all the rules and restrictions that the system imposes on specific data will be met, otherwise the transaction will not be completed and the data in the system will return to its previous state. I - Isolation - isolation is a property that says that during the execution of a transaction, parallel transactions should not affect its result. This property is resource-intensive, so it is typically implemented in part by allowing certain levels of insulation that solve certain insulation problems. We will discuss this in more detail in the next question.
82. What are the transaction isolation levels?
As I said earlier, providing ACID isolation is a resource-intensive process. Therefore, this property is partially satisfied. There are different levels of isolation, and the higher the level, the greater the impact on productivity. Before moving on to transaction isolation levels, we need to look at the various problems of insufficient transaction isolation :-
phantom reading - when the same sample (the same query) is repeatedly called within the same transaction, the received data differs, which occurs due to data insertions by another transaction;
-
non-repeating reading - when the same sample (the same query) is repeatedly called within the same transaction, the received data differs, which occurs due to changes (update) and deletions of data by another transaction;
-
dirty read - the process of reading data added or changed by a transaction that is subsequently not confirmed (rolled back), i.e. reading invalid data;
-
lost update - when different transactions change the same data at the same time, all changes except the last one are lost (reminiscent of the “race condition” problem in a multi-threaded environment).
Isolation level | Phantom reading | Non-repetitive reading | Dirty reading | Lost update |
---|---|---|---|---|
SERIALIZABLE | + | + | + | + |
REPEATABLE READ | - | + | + | + |
READ COMMITTED | - | - | + | + |
READ UNCOMMITTED | - | - | - | + |
NONE | - | - | - | - |
83. What is the difference between Statement and PreparedStatement?
And here there is not a very smooth transition to the features of JDBC technology . So, first, let's figure out what Statement actually is . This is an object that is used to generate SQL queries. JDBC uses three types - Statement , PreparedStatement and CallableStatement . We won’t look at CallableStatement today: let’s talk about the difference between Statement and PreparedStatement .-
Statement is used to execute simple SQL queries without incoming, dynamically inserted parameters. PrepareStatement is used with the ability to dynamically insert input parameters.
-
To set parameters in PreparedStatement, input parameters in the request are written as question marks, so that a value can then be inserted instead using various setters, such as setDouble() , setFloat() , setInt() , setTime() .... As a result, you won't insert the wrong type of data into your query.
-
PreparedStatement is “precompiled” and uses caching, so its execution can be slightly faster than querying from Statement objects . As a result, SQL queries that are executed frequently are written as PreparedStatement objects to improve performance .
-
Statement is vulnerable to SQL injections, while PreparedStatement prevents them. Read more about eliminating SQL injections and other best practices in Java security in this article .
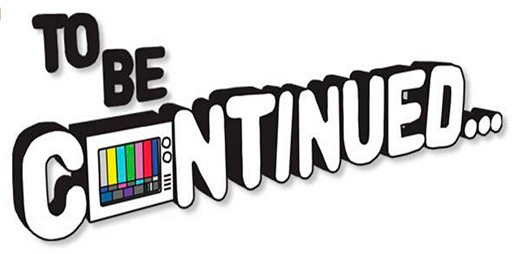
GO TO FULL VERSION